Create Selenium Python script: login form with a wrong password
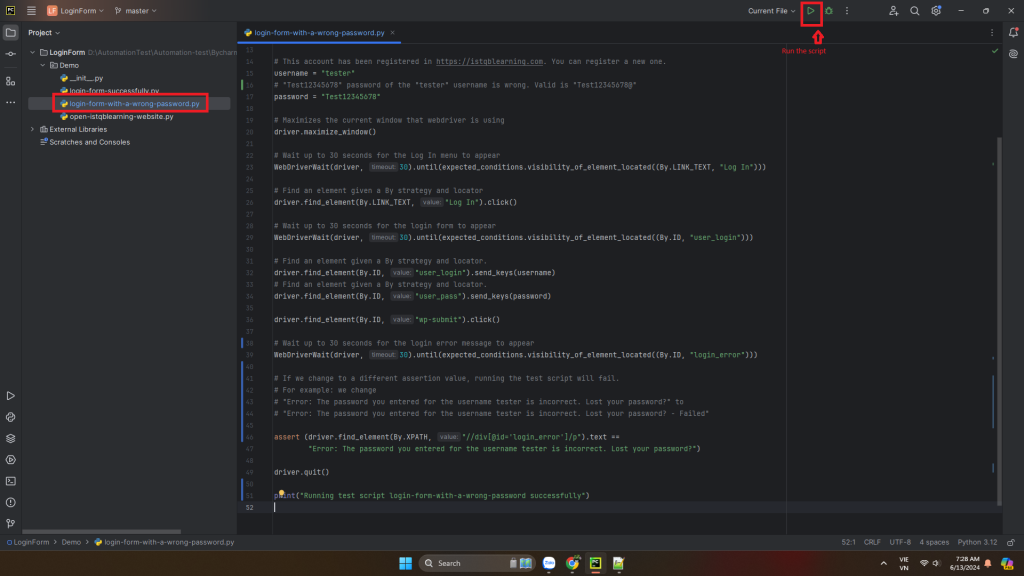
Copy and past the script below into login-form-with-a-wrong-password.py file.
from selenium.webdriver.common.by import By
from selenium import webdriver
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.wait import WebDriverWait
# Creates a new instance of the chrome driver.
driver = webdriver.Chrome()
# Loads https://istqblearning.com web page in the current browser session
driver.get("https://istqblearning.com")
# Wait up to 30 seconds for the website to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Home")))
# This account has been registered in https://istqblearning.com. You can register a new one.
username = "tester"
# "Test12345678" password of the "tester" username is wrong. Valid is "Test12345678@"
password = "Test12345678"
# Maximizes the current window that webdriver is using
driver.maximize_window()
# Wait up to 30 seconds for the Log In menu to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Log In")))
# Find an element given a By strategy and locator
driver.find_element(By.LINK_TEXT, "Log In").click()
# Wait up to 30 seconds for the login form to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.ID, "user_login")))
# Find an element given a By strategy and locator.
driver.find_element(By.ID, "user_login").send_keys(username)
# Find an element given a By strategy and locator.
driver.find_element(By.ID, "user_pass").send_keys(password)
driver.find_element(By.ID, "wp-submit").click()
# Wait up to 30 seconds for the login error message to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.ID, "login_error")))
# If we change to a different assertion value, running the test script will fail.
# For example: we change
# "Error: The password you entered for the username tester is incorrect. Lost your password?" to
# "Error: The password you entered for the username tester is incorrect. Lost your password? - Failed"
assert (driver.find_element(By.XPATH, "//div[@id='login_error']/p").text ==
"Error: The password you entered for the username tester is incorrect. Lost your password?")
driver.quit()
print("Running test script login-form-with-a-wrong-password successfully")
Step | Description |
1 | Opens a Chrome browser |
2 | Loads the https://istqblearning.com/ website from the current browser |
3 | Waits up to 30 seconds for the website to display successfully |
4 | Maximizes the website window |
5 | Waits up to 30 seconds for the Log In menu to appear |
6 | Clicks on Log In menu of the website |
7 | Waits up to 30 seconds for the Login form to appear |
8 | Inputs username into Username or Email Address textbox |
9 | Inputs an invalid password into Password textbox |
10 | Clicks on Log In button on the login form |
11 | Wait up to 30 seconds for the login error message to appear |
12 | Assert the “Error: The password you entered for the username tester is incorrect. Lost your password?” message. If running the test script without any error, the behavior of logging works as expected. |
13 | Quits the current browser |
14 | Print the message in console “Running test script login-form-with-an-invalid-password successfully” |
Here is the statement of the step 12
assert (driver.find_element(By.XPATH, "//div[@id='login_error']/p").text ==
"Error: The password you entered for the username tester is incorrect. Lost your password?")
What is XPATH in Selenium?
A HTML document can be considered as a XML document, and then we can use xpath which will be the path traversed to reach the element of interest to locate the element. The XPath could be absolute xpath, which is created from the root of the document. Example – /html/form/input[1]. This will return the male radio button. Or the xpath could be relative. Example- //input[@name=‘fname’]. This will return the first name text box.
Selenium provides support for these 8 traditional location strategies in WebDriver. This https://www.selenium.dev/documentation/webdriver/elements/locators/ link explains all of them in details.
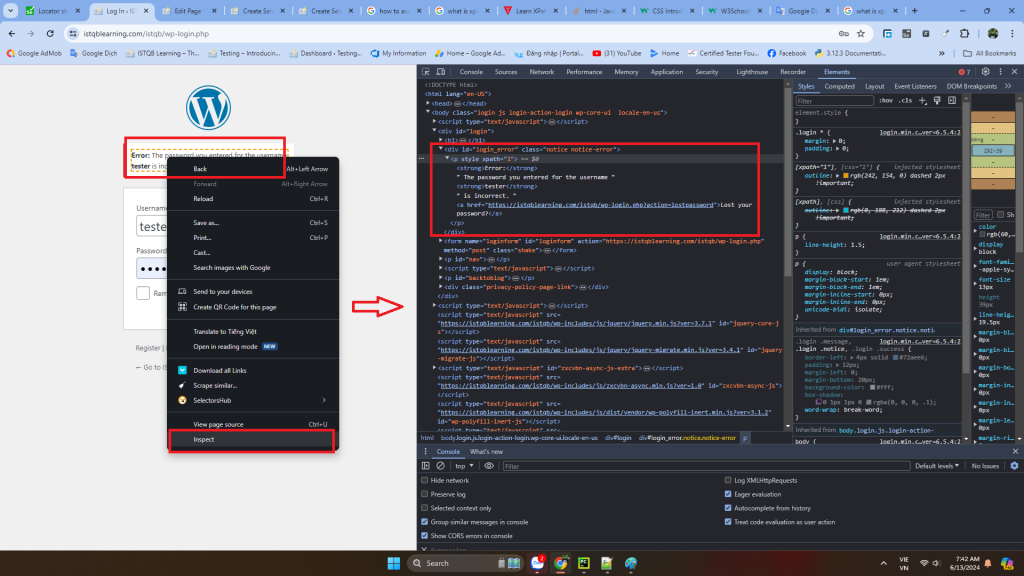
In this step, we use the Inspect feature of Chrome browser to get a value of an element in a website.