Create Selenium Python script: login form successfully
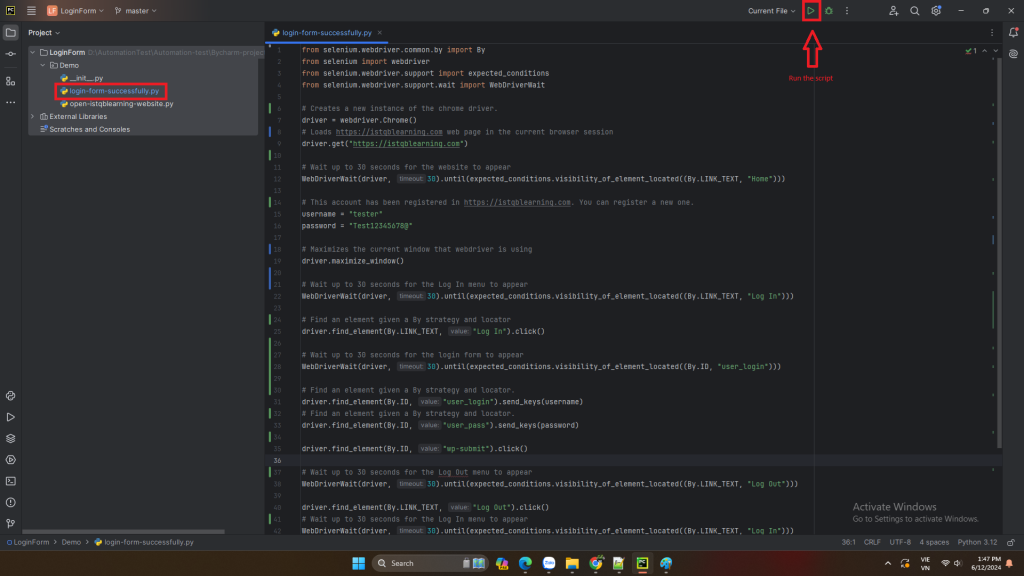
Copy and past the script below into login-form-successfully.py file.
from selenium.webdriver.common.by import By
from selenium import webdriver
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.wait import WebDriverWait
# Creates a new instance of the chrome driver.
driver = webdriver.Chrome()
# Loads https://istqblearning.com web page in the current browser session
driver.get("https://istqblearning.com")
# Wait up to 30 seconds for the website to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Home")))
# This account has been registered in https://istqblearning.com. You can register a new one.
username = "tester"
password = "Test12345678@"
# Maximizes the current window that webdriver is using
driver.maximize_window()
# Wait up to 30 seconds for the Log In menu to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Log In")))
# Find an element given a By strategy and locator
driver.find_element(By.LINK_TEXT, "Log In").click()
# Wait up to 30 seconds for the login form to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.ID, "user_login")))
# Find an element given a By strategy and locator.
driver.find_element(By.ID, "user_login").send_keys(username)
# Find an element given a By strategy and locator.
driver.find_element(By.ID, "user_pass").send_keys(password)
driver.find_element(By.ID, "wp-submit").click()
# Wait up to 30 seconds for the Log Out menu to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Log Out")))
driver.find_element(By.LINK_TEXT, "Log Out").click()
# Wait up to 30 seconds for the Log In menu to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Log In")))
driver.quit()
print("Log in and log out the https://istqblearning.com website successfully")
Step | Description |
---|---|
1 | Opens a Chrome browser |
2 | Loads the https://istqblearning.com/ website from the current browser |
3 | Waits up to 30 seconds for the website to display successfully |
4 | Maximizes the website window |
5 | Waits up to 30 seconds for the Log In menu to appear |
6 | Clicks on Log In menu of the website |
7 | Waits up to 30 seconds for the Login form to appear |
8 | Inputs username into Username or Email Address textbox |
9 | Inputs password into Password textbox |
10 | Clicks on Log In button on the login form |
11 | Waits up to 30 seconds for the Log Out menu to appear. It means user has been logged successfully |
12 | Clicks on Log out menu of the website |
13 | Waits up to 30 seconds for the Log In menu to appear. It means user has been logged out successfully |
14 | Quits the current browser |
15 | Print the message in console “Log in and log out the https://istqblearning.com website successfully” |
How to find out details of an element in a web page? We can use the Chrome browser’s Inspect feature
For example, at the step 3 of the scenario. We can check any element in the home page. In this case we will check Home element.
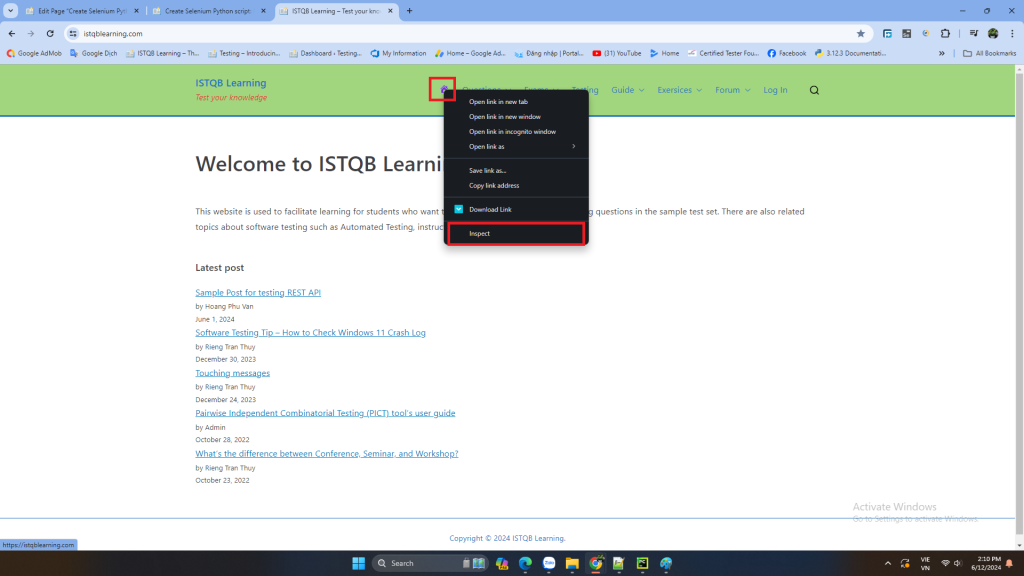
Right click on Home icon and the select Inspect feature.
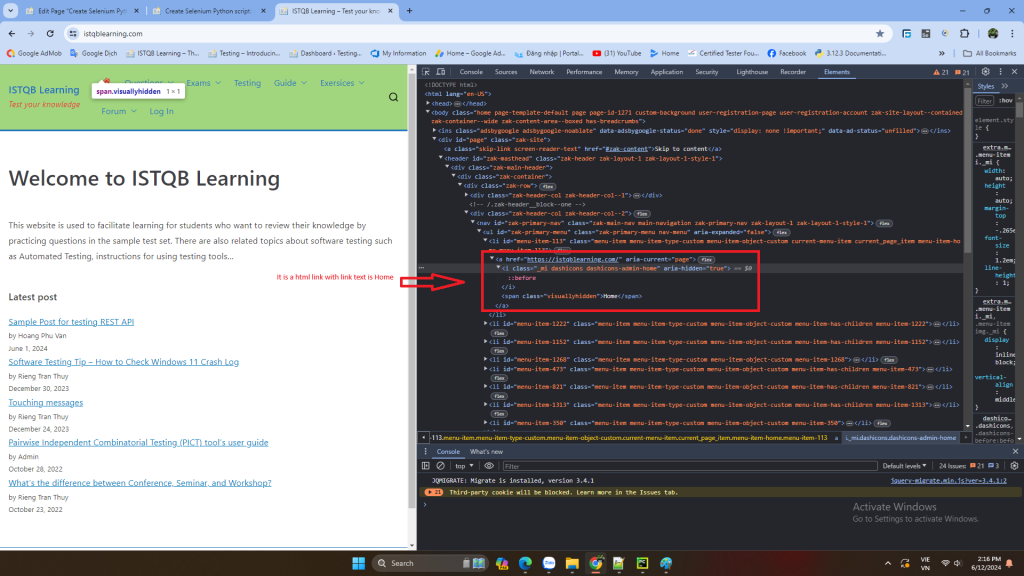
Here is the statement of the step 3
# Wait up to 30 seconds for the website to appear
WebDriverWait(driver, 30).until(expected_conditions.visibility_of_element_located((By.LINK_TEXT, "Home")))
What is the link text locator? Selenium provides support for these 8 traditional location strategies in WebDriver. This https://www.selenium.dev/documentation/webdriver/elements/locators/ link explains all of them in details.
Similar to the other steps in the scenario. We can use this method to their statement.